How to use BitBucket Server APIs to get file contents?
Setting the context
BitBucket (BB) is an Atlassian product, a git-based source control repository. It has a user-friendly interface that allows users to view their repositories, commits, pull requests, anything and everything related to source code changes. The tool is available as a Server edition as well as available on Cloud. There are various ways to work with the tool –
- Direct UI = users can directly work with the interface
- Utilities = It is rare that users will directly work on the UI, it is not recommended as well. Developers use utilities like Sourcetree or Git Bash or Visual Code (VS Code) to clone BB repos and work locally, the recommended best practice.
- APIs = The tool offers an excellent set of APIs that allows developers to retrieve or perform actions based on some criteria.
Scenario
A developer needs to retrieve file contents from BB Server repository and publish it – destination could be any other platform that will be processing this information. There are APIs available that can be leveraged; for this scenario let us look on how to use such an API with Python scripting.
Python for accessing BitBucket Server APIs to get file content
Before you start, it is important that you also define the authentication method for getting file contents. While OAuth can be used, for this example we will leverage a token from BB.
Pre-requisites:
Login into BB, and generate a token that will be used in the Python script to authenticate your access on the required file/s. Access BB and switch to the “Manage account” link from the top right corner as shown below and then click on the option “HTTP access tokens” option (this will generate a Personal Access Token-PAT)-
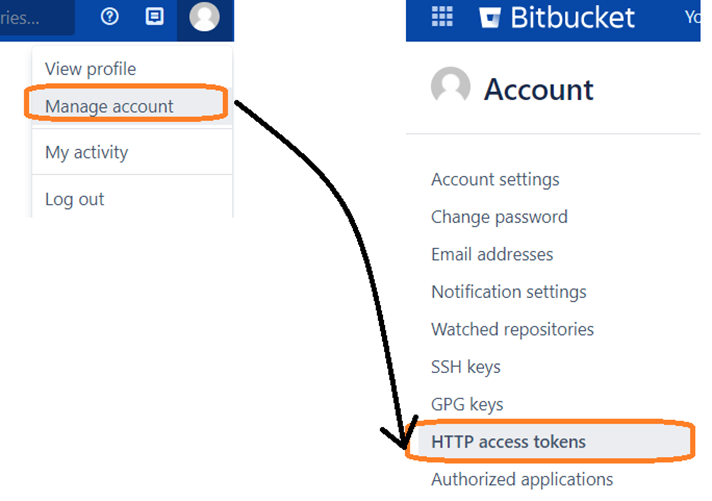
Click on the button on the right side “Create token”, give it a name; and the tool will generate a token value that you will need to save (this is the value you will refer when accessing APIs)-
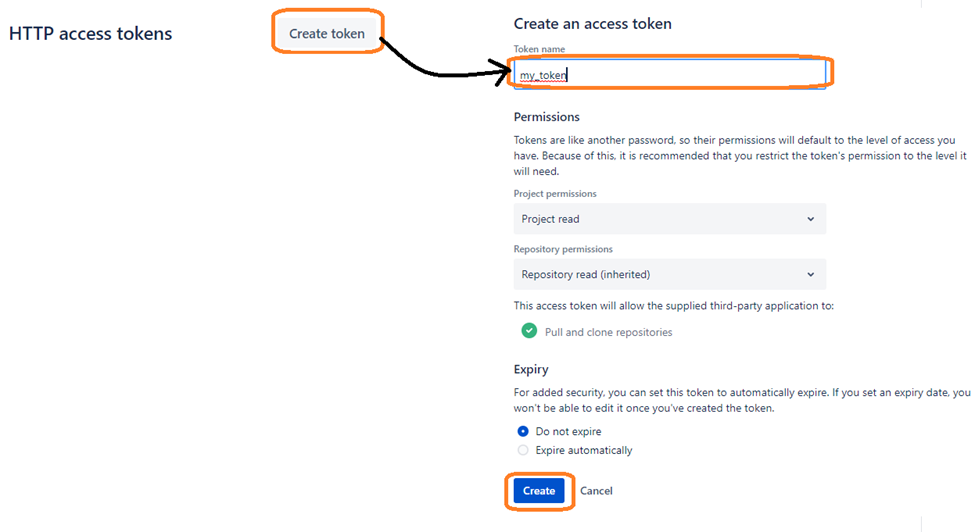
By default, the token has read only access, you can change as needed. For our scenario, we just need read permissions. The tool will show you a token value – do not miss to save that.
BitBucket Server API for reading file content (v1.0)
Python in Action
To script this scenario, am using “Eclipse IDE for Eclipse Committers 2021-03” edition that is enabled Python. Open a new script and type in the following script-
import requests
'set the token value, the one created in BB'
token: str = (
f"Bearer tokenvaluefrombb"
)
'define header content'
headers = {"Content-Type": "application/json", "Authorization": token}
'make a call to the API and '
get_content=requests.get("https://<<bitbucketserveraddress>>/rest/api/1.0/projects/<<projectname>>/repos/<<reponame>>/browse/<<filename>>?raw", headers=headers, verify=False)
print("display file content")
print(get_content.json())

There are five things you need to update in this file-
- <<tokenvaluefrom bb>> : the one you get from BB (refer the pre-requisites section above)
- <<bitbucketserveraddress>> : your server name (the one you use to open the BB UI
- <<projectname>> : mention the project name which holds the repository whose file content is to be retrieved
- <<reponame>> : the repository that has the file to be retrieved
- <<filename>> : the file name that has to be read. If the file resides in a folder, do mention the same as “foldername/file name”.
Save the above script and run it to view file contents. The API returns values in JSON format, hence the variable “get_content” that receives the file contents is displayed with “.json()” function. The content is saved in a variable as a dict object. You can further refine the content as needed.