Welcome back!! Our last chapters focused on PowerShell scripting (Chapter 1, Chapter2) and how we can call or include them in an Azure DevOps pipeline (Chapter 3). In this chapter, we will talk about how to call REST APIs in PowerShell. For example, we need to a run a PowerShell script that contacts a web server, retrieves required information and displays it. To access that server, we need an authorized mechanism to communicate and exchange information. And this is where REST APIs come to rescue.
REST refers to Representational State Transfer Technology – a light weight approach for communication (meaning it consumes less bandwidth) usually used in web services development. APIs refer to Application Programming Interface – refers to the program that performs the tasks between applications or say two platforms. REST APIs, also known as RESTful APIs are now widely used as an easy way to communicate between systems or communicate with cloud services.
For using REST APIs in PowerShell, we need to understand two commands-
- Invoke-WebRequest, and
- Invoke-RESTMethod
Both the above commands send and receive data in HTTP format except that the latter has built-in JSON parsing capabilities, authentication capabilities and returns PowerShell objects that are easy to interpret.
EXAMPLE
To check out on how to use REST APIs – lets assume that we have a tool like Qualys, and we have APIs available to retrieve information from this tool. Qualys is an excellent security testing tool that detects for vulnerabilities on various assets that can be VMs, servers, workstations, etc. We will use the “Invoke-RESTMethod” to call the related APIs provided by Qualys.
Pre-requisites: Manager level access for API execution in Qualys
Step 1 -> Open PowerShell ISE application
Step 2 -> Type in the below script –

Click here to view the script (NOTE- Copy and save the script on your machine with name “view-reports.ps1” and then open this file in your PowerShell ISE application. Do mention your username and password against the variables $username and $password).
Step 3 -> Run this “view-reports.ps1” script – it displays the scans done in 2020
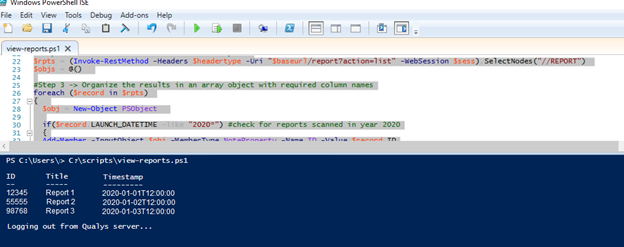
UNDERSTANDING THE INVOKE-RESTMETHOD
There are 2 Qualys APIs that are referred in the above script, lets understand the flow-
Invoke-RESTMethod
-Headers <<mention powershell type>>
-Uri <<mention the web URL to call with API function name>>
-Method <<mention HTML method type which is ‘Post’ in this case>>
-Body <<mention username and password, called when session API is called>>
-SessionVariable <<session variable setup once>>
-WebSession <<session variable to be referred>>
UNDERSTANDING THE SCRIPT
Step (a) –> We define variables $username, $password, $headertype, $baseurl and $body
Step (b)–> We call the first REST API “/session” that authenticates the user and sets up a session. If the session isn’t setup then an error will be displayed and the script will end else it will proceed to next step.
Step (c) –> We then call the next REST API “/report” that extracts the list of scanned reports by mentioning the “action=list” and stores the list in the variable $rpts
Step (d) –> Scan each record, check whether the scan was performed in 2020 and organize the results in $objs variable
Step (e) –> Display the tabular data – it displays the Report ID, Report Title and Date/Time
Step (f) –> Logout from the session and end the script
Try using Invoke-RESTMethod with other API calls.
Happy Scripting !!