In our earlier post introduced the Google Cloud SDK and how using PowerShell we can retrieve list of VMs (Compute Engine) from Google Cloud. Now lets explore other “gcloud” commands in PowerShell.
Pre-requisites: Google SDK is installed and there is an authorized connectivity to the targeted GCP instance (like discussed in earlier post, click here to view the same).
Scenario 1: Display Compute Engines/VM names that are in “RUNNING” state in tabular format. Also display a custom message in case no such VM exits. The following code will help in implementing this scenario-
$result=gcloud compute instances list –filter=”status=RUNNING” –format “[box]”
if(!$result)
{
echo “Unable to find the VMs in RUNNING state” #display custom message
}
else
{
echo “Found RUNNING VMs-“
echo $result
}
Let us save the above code and call the file as “checkVM.ps1” and run this script. The following output will be displayed (assuming there is just 1 VM that exists on GCP instance BUT currently it is not in RUNNING mode)-
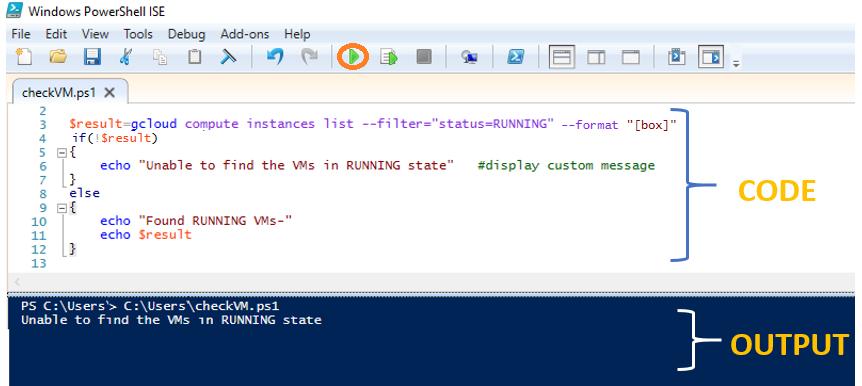
Now, lets change the state of this VM on GCP to RUNNING state and then re-run this script again. The following output will be displayed-
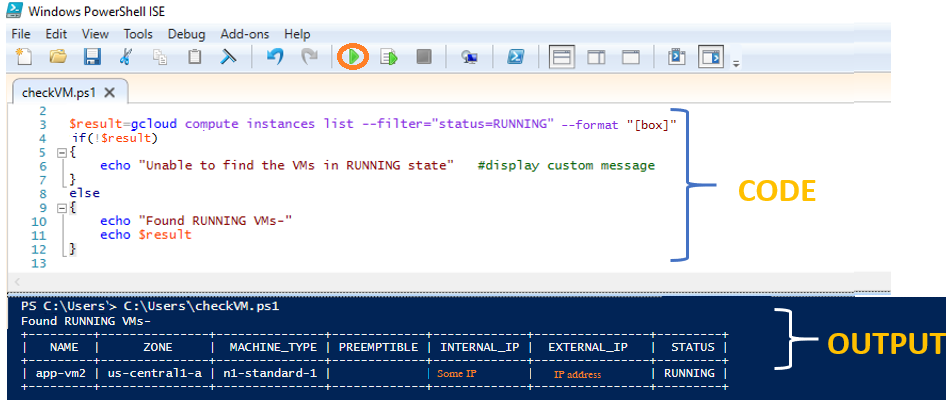
Scenario 2: Display disk volume for a VM/Compute Engine named “app-vm2”. Let us create a file named “checkVMDisk.ps1” and type in following code-
$result_disk=gcloud compute disks list –filter=”app-vm2″
echo $result_disk
Let us run this file and view the disk space details for “app-vm2” VM/Compute Engine. The output shows that the mentioned VM has 10 GB disk.
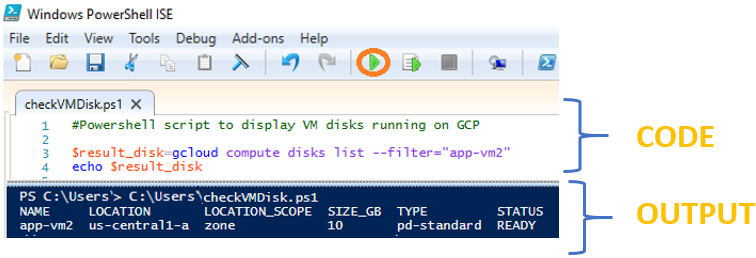
Scenario 3: Display network and subnet information. Create a new file, type in below code and save the file with name “checkNetworkDetails.ps1“-
$result_network=gcloud compute networks list
echo $result_network
$result_subnet=gcloud compute networks subnets list
echo $result_subnet
Now, lets run this file to view results as shown below. The output shows the network details for mentioned VM as well the subnet ranges-
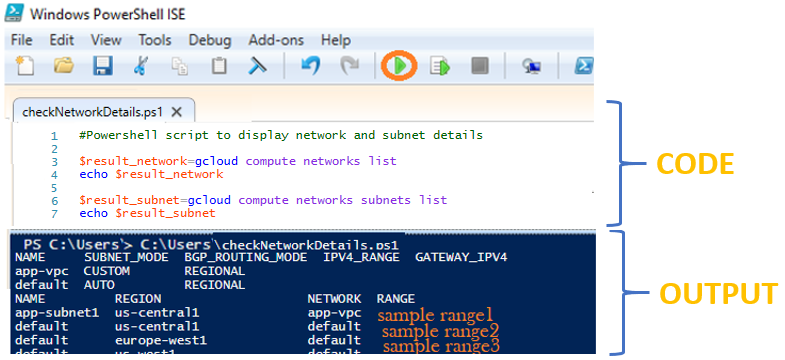
Please note– For each of the mentioned scenarios above, you can display the output in JSON format. For example if we want to view the running VMs in JSON format instead of tabular (box) format, refer the below commands-
$result=gcloud compute instances list –filter=”status=RUNNING” –format “json”
Wasn’t that simple? 🙂 That’s it for now but keep watching this space for more details on exploring GCloud commands and creating complex scripts.